난수(랜덤 넘버) 생성은 프로그래밍에서 자주 사용되는 기능입니다.
확률을 적용하기 위해 난수를 사용할 수 있습니다.
로또 번호를 생성하는 프로그램을 작성할 때 난수 생성 방법을 활용할 수 있습니다.
자바에서 랜덤 넘버를 생성하는 방법을 알아보겠습니다.
1. Math.random()
자바에서는 기본적으로 Math 클래스의 random()으로 랜덤 값을 만들 수 있습니다.
Math.random()은 0과 1 사이(0.0 ≤ x < 1.0)의 실수(double) 값을 리턴합니다.
Math.random로 특정 범위의 값을 생성하려면 아래 공식을 사용하면 됩니다.
Math.random() * (max - min + 1) + min
min과 max 사이의 double 값 중 한 개를 생성할 수 있습니다.
정수 값을 원하는 경우 int로 형 변환하면 min ≤ x ≤ max 사이의 값을 생성할 수 있습니다.
(int) (Math.random() * (max - min + 1) + min)
Math.random() 예제 코드는 다음과 같습니다.
public class RandomGenerator {
public static void main(String[] args) {
System.out.println("0.0 <= x < 1.0 (double): " + Math.random());
int min = 5, max = 10;
System.out.println("5.0 <= x <= 10.0 (double): " + (Math.random() * (max - min + 1) + min));
System.out.println("5 <= x <= 10 (int): " + (int) (Math.random() * (max - min + 1) + min));
}
}
다음과 같은 결과를 출력합니다.
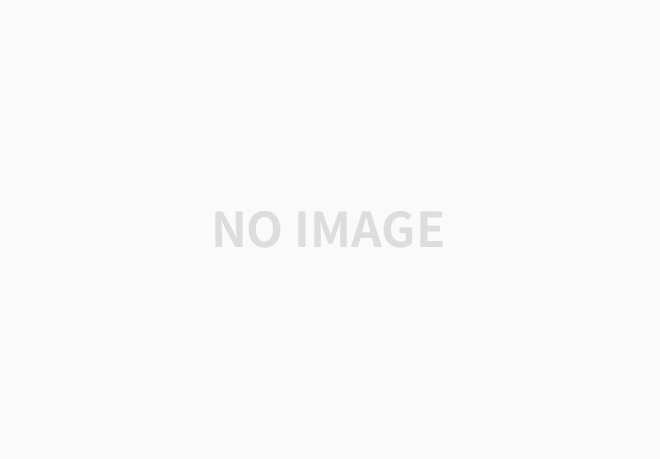
Math.random()은 0과 1사이의 랜덤 넘버만을 생성하는 기능만 제공합니다.
또한 별도로 시드(난수표 선택을 위한 값)를 지정하는 기능이 제공되지 않습니다.
그렇기 때문에 다음에 설명할 Random 클래스를 사용하는 방법을 추천합니다.
2. java.util.Random 클래스
Random 클래스를 사용하는 경우 간단하게 다양한 타입의 난수 생성이 가능합니다.
각 타입의 난수 생성을 위한 메소드들은 다음과 같습니다.
nextBoolean() |
true 혹은 false |
nextInt() |
-2147483648 ≤ x ≤ 2147483647 (Integer) |
nextInt(int bound) |
0 ≤ x < bound (Integer) (bound 값은 포함하지 않음) |
nextLong() |
-9223372036854775808L ≤ x ≤ 9223372036854775807L (Long) |
nextFloat() |
0.0 ≤ x < 1 |
nextDouble() |
0.0 ≤ x < 1 |
nextGaussian() |
평균 0.0이며 표준편차가 1.0인 정규분포(가우스 분포) 난수 생성 |
원하는 타입에 맞춰 제공되는 함수를 사용하면 됩니다.
import java.util.Random;
public class RandomGenerator {
public static void main(String[] args) {
Random random = new Random();
random.setSeed(System.nanoTime());
int bound = 5;
System.out.println("nextBoolean(): " + random.nextBoolean());
System.out.println("nextInt(): " + random.nextInt());
System.out.println("nextInt(bound): " + random.nextInt(bound));
System.out.println("nextLong(): " + random.nextLong());
System.out.println("nextFloat(): " + random.nextFloat());
System.out.println("nextDouble(): " + random.nextDouble());
System.out.println("nextGaussian()" + random.nextGaussian());
}
}
다음과 같은 결과를 출력합니다.
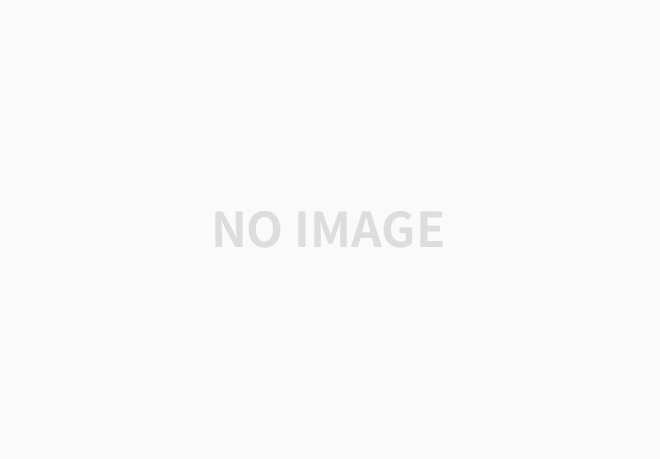
또한 Random 객체 생성시 시드(Seed)를 설정할 수 있습니다.
난수 생성시 기본적으로 현재 시간을 시드로 사용하지만 별도의 시드를 지정할 수 있습니다.
생성자에 입력해서 생성하거나 setSeed()로 설정할 수 있습니다.
3. 로또 번호 생성 예제
로또 번호 생성은 난수 생성을 위한 최적의 예제 중 하나입니다.
Random.nextInt(bound)를 활용하면 로또 번호를 생성할 수 있습니다.
다만 nextInt(bound)의 경우 0부터 시작해서 bound를 포함하지 않기 때문에 아래의 공식을 활용하면 됩니다.
(Math.random() * (max - min + 1) + min)
nextInt()는 int 타입을 리턴하기 때문에 형 변환을 할 필요가 없습니다.
다음 예제는 로또 번호 생성을 위한 코드입니다.
import java.util.Random;
import java.util.Set;
import java.util.TreeSet;
public class RandomGenerator {
public static void main(String[] args) {
Random random = new Random();
random.setSeed(System.nanoTime());
int min = 1, max = 46;
Set<Integer> result = new TreeSet<>();
while (result.size() != 6) {
result.add(random.nextInt(max - min + 1) + min);
}
for (int number : result) {
System.out.println(number);
}
}
}
Set을 사용해서 1과 46 사이에서 중복되지 않는 6개의 숫자를 랜덤하게 생성하는 코드입니다.
다음과 같이 6자리의 로또 번호가 랜덤하게 생성되는 것을 확인할 수 있습니다.
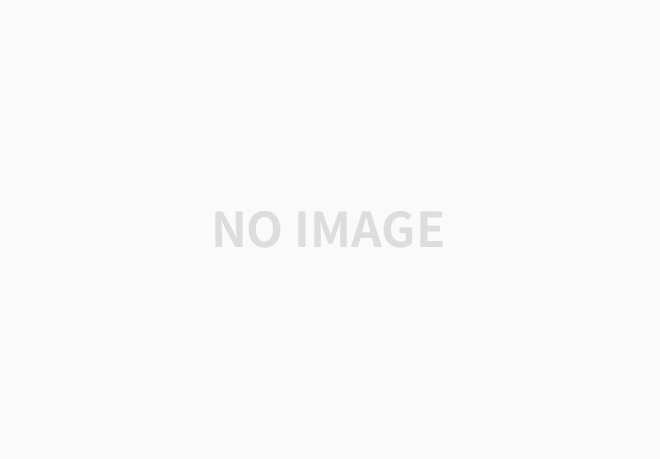
자바의 Random은 간단하게 사용이 가능하기 때문에 난수 생성이 필요한 경우 쉽게 사용이 가능합니다.
'Programming > Java' 카테고리의 다른 글
[Java] 자바 Vector 사용 방법 (0) | 2021.04.06 |
---|---|
[Java] 자바 LinkedList 사용 방법 (0) | 2020.11.28 |
[Java] 자바 ArrayList 사용 방법 (0) | 2020.11.11 |
[Java] BigInteger로 for loop (반복문) 적용 (0) | 2020.09.17 |
자바(Java) JDK 설치 및 환경 변수 설정 (0) | 2018.11.13 |